ID : 789
Detect Function
Detect function is used to save robot coordinates at the time I/O signals are input.
I/O signals that can be input are Hand I/O or MiniI/O input signals. However, MiniI/O exclusive input signals cannot be specified.
For robot coordinates, select position type, joint type, or homogenous transform type data to be stored into global variables.
Executing the "DetectOn" command initializes the buffer of the specified port and enables the Detect function. When the function is enabled, the current position at the time the specified input signal is received is stored into the buffer."Executing the "DetectOff" command stores the data in the buffer into the specified global variable and disables the Detect function.
Each buffer is created separately and identified by a unique port number to edge specification combination.
Function Flow
1
Execute the "DetectOn" command to enble the function.
2
When the specified input signal is detected, position data is stored into the internal buffer.
3
Execute the "DetectOff" command to store data in the buffer into the specified global variable.
Detect Function Sample Program 1
A sample program for Pick & Place motion is shown below. Prior to the pick motion of a circular work, the center position of the work is acquired via the robot arm sensor, and positioning will be corrected as necessary.
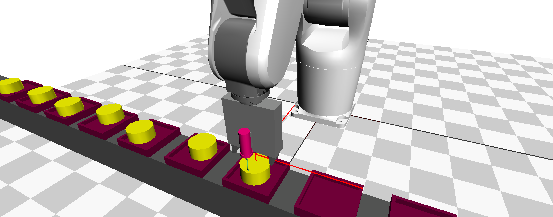
<Pro1.pcs>
#Include "Chuck_Unchuck.pcs"
#Define Home P(200,0,200,180,0,180,-1) 'Home position
#Define SenserToolNum 10 'Sensor's tool number
Sub Main
Dim PickPos As Position 'Work (Pick) position
Dim PlacePos As Position 'Work (Place) position
PickPos = P(300,0,100,180,0,180,-1)
'Approximate position, some misalignment may be present
PickPos = GetCenter(PickPos) 'Position correction program
if PickPos = P(0,0,0,0,0,0,-1) Then Exit Sub
TakeArm
'Pick
Approach P, PickPos, 50
Move L, PickPos
Call Func_Chuck(On) 'Pick
Depart L, 50, Speed = 50
'Place
Approach P, PlacePos, 50, Speed = 50
Move L, PlacePos, Speed = 50
Call Func_Chuck(Off) 'Place
Depart L, 50
Move P, Home
End Sub
Function GetCenter(ByVal pPos As Position) As Position
'Argument "pPos" is the approximate reference position (on the circular work).
'Motion range is +/-100 mm in the X and Y axis directions from this position.
'Trigger I/O is port 128.
'Storing variable for acquired data: I[10]
Dim pGloNum As Integer = 10
'P type global variable number for sensor position aquisition
Dim xPos As Single
Dim yPos As Single
Dim LastPos As Position 'Store previous position
Dim LastToolNum As Integer 'Store previous tool number
LastPos = CurPos
LastToolNum = CurTool
I[10] = 0 'Reset storing variable for acquired data
TakeArm
ChangeTool SenserToolNum 'Change to sensor's tool coordinates
'Sensing in the X direction
Move P, Dev(pPos, P(-100,0,0))
DetectOn 128, 257, pGloNum, 2, 10, 2 'Detect function ON
Draw L, V(200,0,0), Speed = 10 'Move in the X direction
DetectOff 128, 2 'Detect function OFF
If I[10] <> 2 Then GoTo NotSense 'Abort if data could not be acquired
xPos = 0.5 * (PosX(P[pGloNum + 0]) + PosX(P[pGloNum + 1]))
'Sensing in the Y direction
Move P, Dev(pPos, P(0,-100,0))
DetectOn 128, 257, pGloNum, 2, 10, 2 'Detect function ON
Draw L, V(0,200,0), Speed = 10 'Move in the Y direction
DetectOff 128, 2 'Detect function OFF
If I[10] <> 2 Then GoTo NotSense 'Abort if data could not be acquired
yPos = 0.5 * (PosY(P[pGloNum + 0]) + PosY(P[pGloNum + 1]))
'Return to original state
ChangeTool LastToolNum
Move P, LastPos
LetX pPos = xPos 'Substitute x
LetY pPos = yPos 'Substitute y
GetCenter = pPos
Exit Function
NotSense: 'Return initial value when not sensed
GetCenter = P(0,0,0,0,0,0,-1)
End Function
Detect Function Sample Program 2
A sample program is shown below, where the robot arm sensor first detects whether a work is present, and then proceeds with the pick motion.
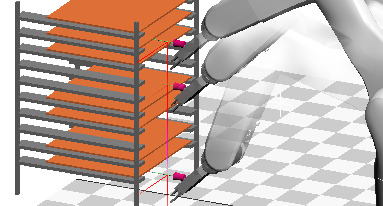
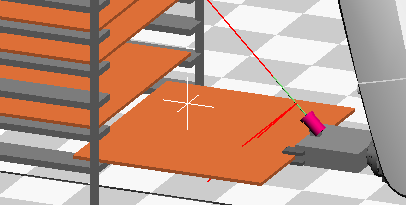
<SearchAndPickPlace.pcs>
'!TITLE "Detect function sample program"
#Define CountIValNum 3
#Define WorkPosPValNumStart 20
#Define pcsPosition 257
#Define SenserIONum 128
#Define MaxCount 10
#Define ScanStartPos P(800.00, 0.00, 50, -104.02, 90.00, -104.02, 5)
#Define ScanEndPos P(800.00, 0.00, 600, -104.02, 90.00, -104.02, 5)
#Define SenserToolNum 3
#Define HandToolNum 4
#Define Home P(466.64, 0.00, 558.33, 100.47, 90.00, 100.47, -1 )
#Define PlacePos P(0.00, 800.00, 100.00, 42.59, 90.00, 133.40, 1 )
'#Include "Chuck_Unchuck"
Sub Main
Dim n As Integer 'Counter
Takearm
Move P, Home
' SCAN
Changetool SenserToolNum
Move P, ScanStartPos
DetectOn SenserIONum, pcsPosition, WorkPosPValNumStart, MaxCount, CountIValNum
Move L, ScanEndPos,speed = 10
DetectOff 128
'If scan could not be performed correctly,
If i[CountIValNum] = -1 Then GoTo ScanErr
'move to each position that was scanned successfully to perform Pick & Place.
For n = WorkPosPValNumStart To (WorkPosPValNumStart + i[CountIValNum] -1)
Call PickAndPlace(p[n])
Next
Exit Sub
ScanErr: 'Process when scan could not be performed correctly
'Statements
End Sub
Sub PickAndPlace(ByVal TargetPos As Position)
Dim LastToolNum As Integer
LastToolNum = CurTool
Dim LastWorkNum As Integer
LastWorkNum = CurWork
TakeArm
ChangeTool HandToolNum
' PICK
Approach p, TargetPos, 30
Move L, TargetPos, Speed = 20
Call FuncChuck(On)
Depart L, 350 , Speed = 20
' PLACE
Move L, PlacePos
Call FuncChuck(Off)
Depart L, 30 , Speed = 20
ChangeTool LastToolNum
ChangeWork LastWorkNum
Move P, Home
End Sub
ID : 789